A reveal effect is where an image on top is erased to reveal another image below it.
This can be used for a simple scratch-off mini-game or a more complicated spotlight or flashlight effect.
There are multiple ways to achieve this effect in Phaser 3 and in this article, we will look at using a RenderTexture
as an alpha mask.
If your reveal effect is fairly simple then you may be able to use a simpler method discussed here.
How it Works
The basic premise is that we will put two images–a cover image and a reveal image–on top of each other and then mask them both with a RenderTexture
.
A RenderTexture
is a texture created at runtime that you can draw into.
A normal Texture
is like an image that we store on our computers. They are created ahead of time in a graphics program while a RenderTexture
does not exist until we run the game and create it in code. It is also empty until we draw into it.
Phaser lets us draw one or more GameObjects
into a RenderTexture
as many times as we want.
Because we are using a RenderTexture
as our alpha mask, the masked area will change as we draw into it. We will be using a brush image–a radial gradient or a transparent image with one dot from a PhotoShop soft brush–to do this.
This process creates the reveal effect.
Setting Up
We will use the Phaser logo as both the cover and reveal images for simplicity. One difference is that the cover image will have a green tint so that we can tell them apart.
Let's set up our Scene.
|
|
Running the Scene now will show a green-tinted Phaser logo.
Nothing too exciting. 😎
Next, let's get to the interesting stuff by creating a RenderTexture
and masking image.
|
|
We make a RenderTexture
on line 29 but do not add it to the Scene. Our RenderTexture
does not need to be visible.
Instead, it will be used as the underlying texture for our maskImage
created on line 35. Note that it is also not added to the Scene.
The maskImage
data will only be used as alpha masks for the 2 Image
instances: reveal
and this.cover
.
Using Alpha Masks
Now that we have all the necessary parts we can create and use a BitmapMask
.
|
|
There are only 3 new lines that you need to worry about.
We create a new BitmapMask
on line 42 and set it to the mask
property of this.cover
.
The mask is then set to invertAlpha = true
because we want to hide the cover image as we draw into our RenderTexture
to show the reveal image. This makes the mask show what should normally hide and hide what should normally show.
Lastly, we create another BitmapMask
and set it to the mask
property of the reveal image and leave invertAlpha
with a default value of false
so that it behaves normally.
This will give us the effect where parts of the reveal image become visible while those same parts become hidden in the cover image.
Drawing into a RenderTexture
The last thing we need to do is handle drawing the brush image into our RenderTexture
when the mouse is pressed and dragged.
|
|
We create an isDown
property on line 12 to keep track of whether the left mouse button is pressed or not.
Then we set this.cover
to interactive and add 3 events: POINTER_DOWN
, POINTER_MOVE
, and POINTER_UP
. The handlers for each are fairly simple. handlePointerMove(pointer)
is where the drawing logic lives.
Next, we create an Image
instance as the brush on line 54 without adding it to the Scene. It will only be used on line 77 to draw into the RenderTexture
.
As the RenderTexture
is updated, the BitmapMask
instances we created on lines 44 and 47 will update and give us a reveal effect.
It will look something like this:
Next Steps
We covered the basic setup for using alpha masks on two different images to create a reveal effect.
This simple alpha mask example should help you get started on more interesting effects.
For example, we will create a spotlight or flashlight effect in the next article. Stay tuned! 👍
Let us know in the comments below if anything doesn’t work or is unclear. We’ll be happy to fix or clarify!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
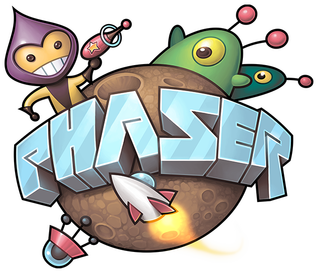