Phaser doesn't come with buttons but making a basic one is easy.
One issue with the basic button is that text can't be added to the button as a child. Phaser is designed to have a flat hierarchy so you can't add children like you might be used to in Flash, cocos2d, or other game frameworks.
Instead, Phaser has a Container
type that is designed to group multiple GameObjects
.
Those coming from Flash or Unity have probably created nodes called “container” to group things for simpler to manage animations.
That is the same concept. In this article, we will create a ButtonContainer
class that inherits from Phaser.GameObjects.Container
to hold a Button
and a Text
object.
Wrapping a Button
The ButtonContainer
class will use a little programming kung-fu to reuse all the logic we implemented in Button
.
First, we will add an IButton
interface to Button.ts
. Then add it to the Button
class definition with implements
. We are using TypeScript so you can ignore this if you prefer plain JavaScript.
|
|
Next, our ButtonContainer
will implement a version of IButton
with two additional methods to handle text.
|
|
Put this in ButtonContainer.ts
.
When we create the ButtonContainer
class we will implement IButtonContainer
which will include all the methods defined in IButton
.
We are doing this so that we can create a Button
in ButtonContainer
and then forward the IButton
method calls to the Button
instance.
This allows us to reuse all the code we already wrote!
ButtonContainer
The ButtonContainer
class will be very simple compared to Button
because we are forwarding most of the heavy lifting to Button
.
The main difference is that we will create a Text
and add it along with the Button
.
|
|
Most of the ButtonContainer
class will be methods that forward to this.button
.
The key differences are in the constructor
where we create a Button
and a Text
and then call this.add()
to add them as children to the ButtonContainer
.
This will allow us to manipulate everything as a single object. 🎉
Augment GameObjectFactory
We can use ButtonContainer
right now by calling new ButtonContainer()
but we can also get a little fancy and augment Phaser's GameObjectFactory
.
This will allow us to use a nicer, idiomatic syntax like: this.add.buttonContainer()
|
|
You can add this to the bottom of ButtonContainer.ts
.
For TypeScript, you'll also want to add a type definition file like types/GameObjectFactory.d.ts
.
|
|
This uses Declaration Merging to provide appropriate IntelliSense and type checking.
Using ButtonContainer
Our Scene is going to be very similar to the one from the basic button.
|
|
The only differences are the highlighted lines!
We import the ButtonContainer
class on line 2 so that Phaser's GameObjectFactory
will be properly augmented with the buttonContainer()
factory function.
Then the button creation code is on lines 32 - 35. We don't need to create a separate Text
object because the ButtonContainer
already creates one.
Run the code and it should look like this:
Next Steps
You now have a button that is more concise to create and easier to animate. From here you can implement more UI elements like checkboxes and radio buttons.
Or you can leverage the fact that Phaser is an HTML5 framework and use the wide selection of CSS UI frameworks like Bootstrap, Bulma, and more!
In the next article, we will show you how to use Phaser's DOMElement
to create HTML buttons that can be controlled from the game code!
Let us know in the comments below if anything doesn’t work or is unclear. We’ll be happy to fix or clarify!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
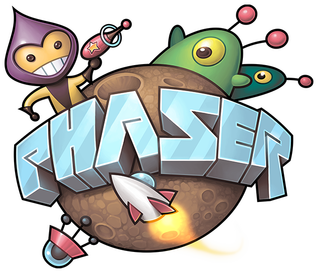