I recently decided to play around with Phaser 3 again with the help of the good people here at Ourcade!
One thing I tasked myself with was to rebuild the Beginning ECS in Phaser 3 tutorial but have it work with ReactJS!
Looking to learn how to use an Entity Component System(ECS) in your Phaser 3 games?
Check out this Ourcade video that uses bitECS–the same library currently being used in the development of Phaser 4.
So before you continue with my tutorial, ensure you understand how the base project was built with Phaser 3, Parcel, and BitECS. I urge you to go check it out if you haven't already.
The aim of this article is to show how you can use the tank game with ReactJS. The details regarding Entity Component System in Phaser has been omitted since it is covered in Beginning ECS in Phaser 3.
Getting Started
Ensure you have node
and npm
installed on your system. Then navigate to your project directory.
|
|
Make sure your folder structure is similar to the screenshot below.
No need for any setup in package.json
as create-react-app
does that for you.
Setup your Phaser Configuration File
|
|
|
|
|
|
Import your Phaser Configuration in the App.tsx
entry file and ensure the div
id is the same as the parent
field in your Phaser Game Config. This allows Phaser to create a canvas
element under your desired div
tag.
|
|
|
|
Now that this has been done you don’t have to worry about anything other than creating your Game scenes, components, and systems.
Setup your scenes in this manner:
|
|
💡 Personal Tip
I always like to create an entry point for my folder where I export all the files in that folder like the example below 👇
|
|
This enables me to import Scenes in any other file in the following format:
|
|
You may notice that I don’t have to add ./scenes/Bootstrap
because it has been specified in ./scenes/index.ts
. It acts as the entry point for all files in the scenes folder.
Now THE GAME Scene
|
|
If you found this helpful please let me know what you think in the comments. You are welcome to suggest changes.
Thank you for reading this article! You can also find the source code on Github.
You can follow me on all my social handles @officialyenum and please subscribe to me on Medium here. 👏 It would would mean a lot!
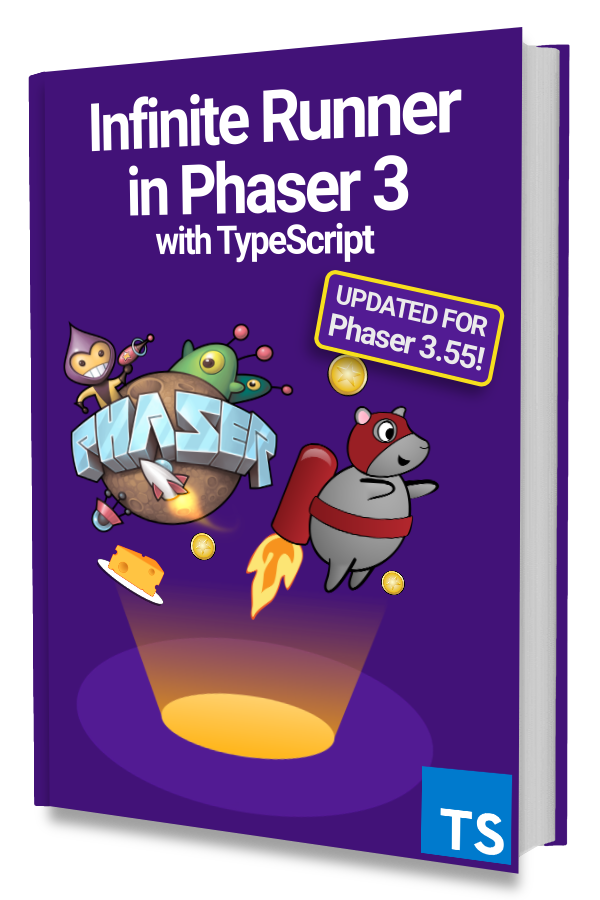