If your game uses a ton of images then preloading everything upfront may not be the best idea.
It could be a collectible card game or something similar where not all players will see every card in the game.
Preloading every possible card is unnecessary or even impossible. At the very least it will make players wait much longer than necessary.
One way to solve this problem is to only load images when they will be shown. This is called lazy-loading and will add a bit of extra complexity for the benefit of a more responsive feeling experience.
We'll show you how to do that in this article by going through a 52 card deck and loading the necessary texture if it hasn't been loaded yet.
Example Set-Up
This example uses the phaser3-typescript-parcel-template and the code examples will be in TypeScript.
If you are not familiar with Phaser 3 and TypeScript then we've got a free book to help you get started!
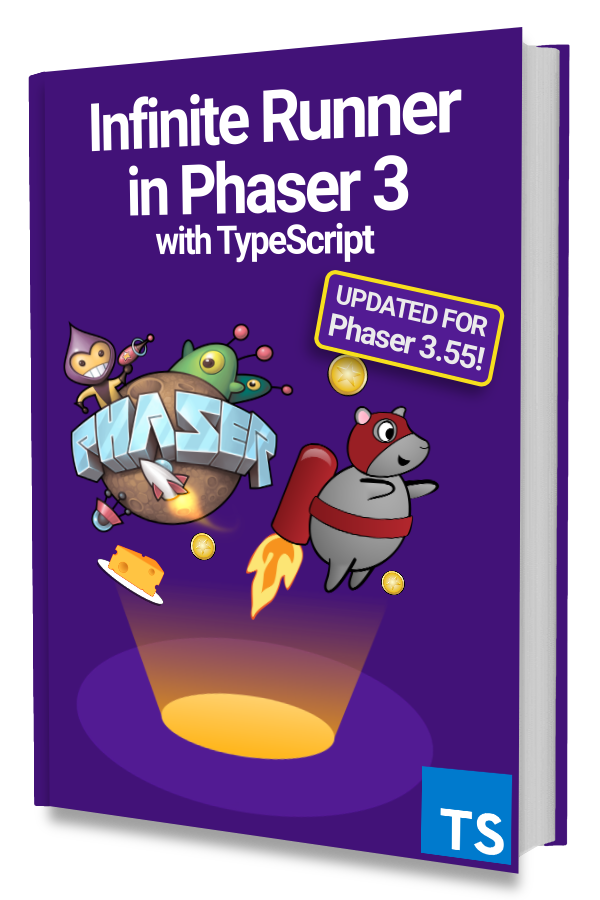
Learn to make an Infinite Runner in Phaser 3 with TypeScript!
Drop your email into the box below to get this free 90+ page book and join our newsletter.
The concepts and approach should work fine if you are using modern JavaScript or a different project set up.
We'll be using a single Scene and a couple of predefined arrays and helper functions 👇
|
|
There is one array that holds the possible card suit values and another with the possible card values from 2 to Ace.
The getCardName()
function creates the file name of an image using the given suit and value arguments.
Then the Scene keeps track of the current suit and value indices so that we can deal out cards in order. The 3 other getter properties (currentSuit
, currentValue
, and currentCardName
) are there for convenience.
Using a Placeholder
We have a preload()
method even though we'll be loading card images dynamically because we want to have a placeholder to use while the real card is being loaded.
This will add to the feeling of responsiveness as the game will continue operating as normal while images are loading in the background.
We'll switch the placeholder card with the real card once it is loaded.
You can use anything you want but we'll simply use a card back in this example 👇
|
|
This means we'll use this 'card-back'
texture when we deal a card that has not been loaded yet.
Deal a Card
Dealing a card is where the majority of the work will be done.
The idea is to figure out which card should be dealt and then checking if the necessary texture has been loaded yet.
If the texture is already loaded then we can just create a card with it.
If the texture is not already loaded then we need to ask the LoaderPlugin
to load it and create a placeholder card.
Then once the card texture we need is loaded we'll swap the placeholder card with the real card.
Here's what it looks like in the deal()
method 👇
|
|
When developing locally you'll barely see the placeholder card so keep that in mind if it looks like nothing is happening. You can also add a delay before setting the card texture to be sure things are working.
Using the Deal Method
For simplicity, we'll use the left and right arrow keys for dealing a new card and removing the last dealt card.
Add this to create()
👇
|
|
The left arrow key will deal a new card and move all existing cards 20 pixels to the left.
Then the right arrow key will remove the last dealt card and move all existing cards 20 pixels to the right.
You'll get something that looks like this 👇
Next Steps
You can use this method for loading any asset that Phaser supports from atlases to audio files.
One way to improve the placeholder graphic is to use a loading animation so that players know something is happening or will change.
Another approach is to predict or forecast what assets the player might need soon and then load them as the game is being played. This would create an even more seamless experience!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
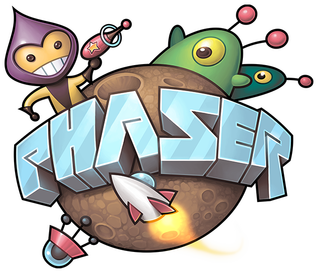