Are you trying to get the Phaser Spine plugin to work but failing miserably at it?
There's a bunch of files in the Github repository but which one do you need? All of them? Just some? And WTF is a .js.map
file?
Being an intrepid developer you've tried your best but all you got were errors like SpinePlugin is not a valid plugin
or this.add.spine is not a function
. ðŸ˜
Shoot me now. 🔫
You are not alone. Many developers learning to use Phaser run into this problem.
We will show you how to get the SpinePlugin to work in a Phaser project that does not use NPM, Yarn, or any web development tooling.
Just your html
and js
files plus a local webserver. If you've gotten far enough to try using the SpinePlugin then you are already running a local webserver.
Here are 5 great options if you are not running a webserver.
You can find the complete source for this example project here.
For using the SpinePlugin with NPM check out this article.
Which Spine Files Do I Need?
Using the right file is the first step that trips up almost everyone.
Nothing else you do will work if you aren't loading the right file.
You only need 1 file and that is the SpinePlugin.min.js
from phaser/plugins/spine/dist
.
If you downloaded Phaser as a zip from Github then find that file from the path above or get it from here.
Order of Script Tags
Now that you have the right file you need to include it in your index.html
.
The order is important so be sure phaser.min.js
is loaded first.
|
|
We are putting our game code in main.js
but you might have something like game.js
instead.
What's important is the specific order: your game code should be last.
Load SpinePlugin into Phaser
We can load the SpinePlugin
by updating the config
object passed to Phaser.Game()
.
|
|
Now in your Scene's preload()
method, you can load Spine animations like this:
|
|
And then create the animation in create()
like this:
|
|
Ugh, It Still Doesn't Work!
If you are one of the lucky people still running into errors then try using the PackFile approach instead.
Remove <script src="SpinePlugin.min.js"></script>
from your index.html
and then update the scene
entry of your GameConfig
like this:
|
|
Notice we also removed the plugins
entry from config
.
Your project likely will have multiple Scenes including a bootstrap or preload Scene. You'll want to add the pack
configuration to the first Scene that loads.
If you are subclassing Phaser.Scene
then add the pack
entry to the Scene SettingsConfig
:
|
|
Next Steps
Now you can use Spine animations in your Phaser game! 🎉
The spineBoy
above is a SpineGameObject
that can be used like other Phaser GameObjects
.
Check out the official documentation to learn how to switch animations, skins, slots, and more.
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
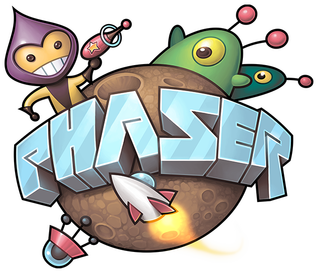