Are you making a top-down game where enemies or opponents should track or keep their eye on the player?
Maybe you have a turret that should face a moving target before firing?
You know that you need to rotate the turret but how do you know what that angle should be?
If you remember your trigonometry classes then you can probably figure it out but Phaser can do the math work for us!
In this article, we will show you how to get that angle using the player's position and the position of the enemy that should face the player.
Angle from Points
You can get an angle from 2 points using Phaser.Math.Angle.Between()
.
That means we just need the player's position and the enemy's position.
Our code to get an angle from those two points would look like this:
|
|
Another way to accomplish the same thing is to use a Vector2
from enemy position to player position like this:
|
|
Either implementation will do what you need but we'll provide a full example with zombies to help you see how it can be used within a Phaser game.
Zombies Example
We are going to put the rotation logic above into a custom Zombie
class so that it will rotate to always look at the player whether the player is stationary or moving.
The code is in TypeScript.
|
|
Our Zombie
extends from Phaser.GameObjects.Sprite
and implements the IZombie
interface that we define. It just requires a setTarget(target: Phaser.GameObjects.Components.Transform)
method.
Then the update(t: number, dt: number)
method should look familiar. We use the resulting rotation
on line 32 with this.setRotation(rotation)
to rotate the Zombie
.
Creating Zombies in a Scene
Our Scene will have 4 zombies placed near each corner and then a 5th one that randomly picks a position.
The player will be represented by a white circle. The keyboard input code to move the player is omitted from the example to focus on the zombies.
|
|
We are using a Group
to let Phaser handle creating and adding new Zombie
instances to the Scene. You can use new Zombie(...)
as well and then manually add them to the Scene.
The important part is on line 42 where we set each zombie's target to be the player.
This is what the end result looks like:
Next Steps
From here you can use distance to determine when a zombie should face and try to attack the player.
In the case of a turret, you can start firing bullets, lasers, or other projectiles at the player.
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
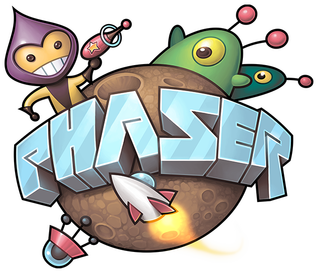