If you are making a space shooter game in Phaser 3 with Arcade Physics and tried to add a homing or target seeking missile then you've probably run into a problem with the physics bounding box.
It doesn't rotate with the GameObject so collisions won't work properly. This is by design
One solution is to use Matter Physics but that could mean changing a lot of existing code. And potentially breaking many things in the process.
There is another option if you can live with a missile that only registers collisions when the tip or front hits a target.
You can find the example project on Github.
Using a Container
This trick uses a Container
with a circle physics body that holds a missile image.
The Container
is never rotated. We rotate the child Image
instead and then the parent Container
is set to move based on where the child Image
is facing.
Using a circle physics body means we can keep the collision area the same no matter how the missile image is rotated.
Remember! This means the missile has to hit targets head-first for a collision to register.
The Missile Container Class
First, take note of the constructor to see how the Image
and circle physics body is setup.
We are using modern JavaScript in this example.
|
|
The origin of the Image
is set to 0.8, 0.5
on line 10. The x
value of 0.8
can be adjusted to accommodate the specific missile graphic and radius size.
A physics body is added to the Container
on line 13.
Then we determine the radius on line 15 and use it to make the body a circle. The multiplier value of 0.3
can be adjusted to best fit the specific missile image.
It is important to offset the Image
by whatever value the radius
is as shown on lines 17 and 18.
Next, the update()
method handles adjusting direction based on target position and movement based on which direction the Image
is facing.
|
|
You can change how nimble and fast the missile is by adjusting the turnDegreesPerFrame
and speed
properties.
Bonus: GameObjectFactory Registration
We can keep our code idiomatic to Phaser by registering our Missile
class with the GameObjectFactory
. This is optional but our usage example will assume this was done.
Add this to the bottom of the Missile
class file.
|
|
Using a Missile
The Missile
class has an update(dt)
method that needs to be called. We can access an update loop from the Scene.
Using the Missile
class from a Scene will look like this:
|
|
The missile is created on line 27 and then mouse location tracking is enabled on the next line.
Notice the call to missile's update(dt)
method from the Scene's update(t, dt)
method.
And that is how you can make a homing or target seeking missile using Arcade Physics! 🎉
Next Steps
Turn on debug mode to make sure everything is working properly in your code. Make this change to your game config:
|
|
Don't miss any future Phaser 3 game development tips, tricks, and knowledge-drops by subscribing to our email list!
Just type in your email into the box below and hit the button!
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
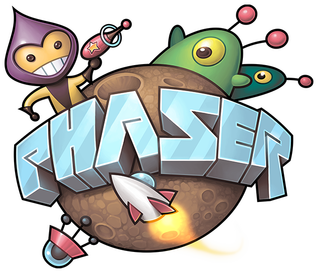