At some point, your game will want text input to collect information from your player like their name for a save file or a high score leaderboard.
Phaser doesn't have native UI controls although you can use any CSS UI framework via the DOMElement
like we did here for buttons.
But maybe you just aren't into HTML. You're a game developer; not a web developer. 💁♀️
If you've done your share of searching in the Phaser community then you've probably come across RexUI by Rex Rainbow.
In this article, we will look at using Rex's Text Edit plugin to let players enter text.
Load the RexUI Plugins
If you are not using npm
then you can load the plugins in the Scene preload
method like this:
|
|
Those URLs might change so consider downloading the files and adding them to your project instead of loading them from Github.
Alternatively, you can use npm
and install the phaser3-rex-plugins
package.
npm install phaser3-rex-plugins
And then add the plugin to your game config:
|
|
Note that we are specifying the id of the container that Phaser should add its canvas
to in the parent
key and setting dom.createContainer
to true
.
We need both these settings because the Rex TextEdit plugin uses an Input element.
Using the TextEdit Plugin
We will need to create a normal Phaser.GameObjects.Text
instance to use with the TextEdit plugin.
The plugin works by hiding the Text
instance and then overlaying an HTML Input element that allows text input.
|
|
The above code should be in the create()
method of your Scene.
The text becomes editable after it is clicked on. The plugin will automatically close on click off or pressing the Enter
key.
Give it a spin and you should be able to edit text after clicking on it. 🎉
Things to Consider
The revealed Input element did not align perfectly with the Phaser Text
instance in our tests so it doesn't look seamless.
A potential solution is to access the Input element and adjust it. The this.rexUI.edit()
method returns a TextEdit
instance.
|
|
We have not tested this approach so your mileage may vary.
Another thing to note is that you can't keep it in edit mode. The player has to click on the text before making edits.
This could be a problem depending on your intended UX and game flow.
Lastly, this is based on the Input DOM element so it will always render above everything else in your game. This is almost never a problem for UI but knowing this might save you hours of trying to layer it between Phaser GameObjects. 😅
Next Steps
Rex has over a dozen other UI components you can use in your Phaser game.
The documentation is not amazing but the general set up is going to look a lot like what we did here for TextEdit.
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques! Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
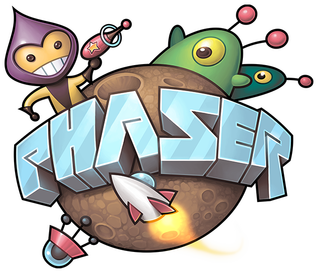
Updated March 17th, 2020: Removed unnecessary plugin imports and onTextChanged
callback after insights from Rex Rainbow.