There are tons of beautiful web fonts we can use for web-based projects–including web-based games–from Google and Adobe.
Phaser is a great framework for making web-based, HTML5 games but it does not handle loading web fonts.
There are a few ways to get web fonts working in your game from hacked together to fully integrated into Phaser as a plugin.
In this article we go just short of making a plugin while still hooking into Phaser's asset management system so that fonts are preloaded before text is created.
We will use the Web Font Loader library along with Phaser's File
class.
Create a WebFontFile
The code here will be in Modern JavaScript but you should be able to adapt it as necessary.
As a best practice fonts should be loaded in the Scene's preload()
method or the text may not render correctly.
We can do that by using this.load.addFile()
which takes a Phaser.Loader.File instance. The File
class is intended as an abstract base class so we will extend it in our WebFontFile
class.
|
|
We are importing WebFontLoader
on line 3. You will have to have run npm install webfontloader
in order for this import to work. Alternatively, you can omit the import and load WebFontLoader
from a CDN.
See the instructions on the WebFontLoader Github page.
Phaser.Loader.File
has several methods you can override but the most important one is load()
. It is where we have WebFontLoader
do its magic. Once the fonts are loaded we let Phaser know on line 27.
In this example, we only implemented support for Google Fonts and will throw an error for any other service.
Note: There are shortcomings to this basic example that we will discuss in the Advanced Example section.
Using WebFontFile in a Scene
Now let's give WebFontFile
a try by preloading a font and creating some text.
|
|
Note: For fonts with spaces in the name we need to include double quotes as you see on line 20.
In preload()
we use this.load.addFile
and pass in a WebFontFile
instance that will load Press Start 2P
from Google Fonts. Then we create text that says Hello World!
with Press Start 2P
as the desired font on line 20 in create()
.
We are using the phaser3-parcel-template to bootstrap our project with a game window size of 800 x 600 so our Scene looks like this:
You can also load multiple fonts by using an array like this:
this.load.addFile(new WebFontFile(this.load, [
'Press Start 2P',
'Lato',
'Roboto'
]))
Advanced Example
The basic WebFontFile
above should work fine in most cases. One thing it does not handle is being used more than once:
this.load.addFile(new WebFontFile(this.load, 'Press Start 2P'))
this.load.addFile(new WebFontFile(this.load, 'Lato'))
Generally, you will want to use an array of names when loading multiple fonts. This allows WebFontLoader
to make a batched request and get all the fonts in one network call.
That said, it doesn't hurt to have a more robust version of WebFontFile
!
|
|
We made changes to use the fontactive
callback instead of active
to check if the specific fonts given in the constructor have been loaded and only say we are finished when that has happened.
The callback change is on line 28 and the logic to check if the specific fonts are loaded starts on line 68 in checkLoadedFonts(familyName)
.
We also hook into fontinactive
because the Internet does not always work and a font may fail to load. Odds are we should continue even though the font is missing. At least the game won't be frozen. 😅
There are more sophisticated ways to handle this problem like retries or fallback fonts but we'll leave that to you. 😎
Lastly, we implemented support for Adobe Edge Web Fonts on lines 42 and 60 - 66. You can use it like this:
this.load.addFile(new WebFontFile(this.load, 'shojumaru', 'adobe-edge'))
Next Steps
If you want to use TypeKit, Fonts.com, or some other service then you need to implement support for them. Just follow the structure described by WebFontLoader
.
You can get a Gist of the advanced WebFontFile example here.
Let us know in the comments below if anything doesn’t work or is unclear. We’ll be happy to fix or clarify!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
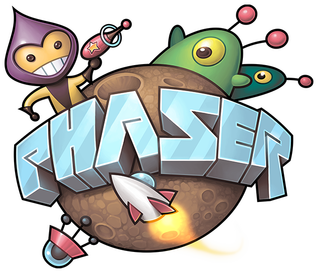