Creating a spotlight or flashlight effect in Phaser 3 is fairly simple once you know how to use alpha masks.
You can also use this technique for a “magic lens” effect or anything that involves revealing hidden information.
If you are not familiar with using alpha masks in Phaser 3 then check out this first.
In this article, we will be building on the code example from our Reveal Effect in Phaser 3 with Alpha Masks to create a flashlight effect that illuminates a darkened Phaser logo.
How it Works
A flashlight effect is fundamentally very similar to a scratch-off effect except you don't do any scratching and the RenderTexture
discards state before being drawn into.
We will use the same setup as we did in the Reveal Effect in Phaser 3 with Alpha Masks article where two Phaser logos were placed on top of each other.
The one on top is the “cover” image and the one below is the “reveal” image. We will tint the cover image a dark blue to give it a night feel.
Our brush will be changed to a simple circle instead of an image to create a stark contrast between lit and unlit. This is just a matter of design and you can use an image if you want.
The effect is created by using alpha masks to hide parts of the cover image that will be shown on the reveal image when the mouse moves over it.
Setting Up the Scene
First, we will create a simple Scene that loads and creates 2 Phaser logo images.
|
|
Everything should be pretty straight forward.
Next, we add a RenderTexture
and masks.
|
|
So far this is the same as what we did in the Reveal Effect in Phaser 3 with Alpha Masks article.
The difference comes in how we handle mouse input and creating the circle that represents light.
Light and Action
We need to draw into our RenderTexture
for the alpha masks to do anything interesting.
So let's create a circle to represent the light from a spotlight or flashlight.
|
|
We create a circle on line 46 with a radius of 30
and alpha of 1
. You can change these values to create a larger, smaller, or dimmer light.
For example, adjusting the alpha value from 1
to 0.5
will make it half as bright.
The light's visible
property is set to false
because it does not need to be displayed. We will only use it to draw into our RenderTexture
.
|
|
We add a listener for the POINTER_MOVE
event on line 50 and handle it in handlePointMove(pointer)
on line 55.
Then on line 60, we clear the RenderTexture
before drawing this.light
into it.
This is a key difference from the scratch to reveal mechanic that keeps all previous changes to the RenderTexture
.
For a light reveal effect we want to make sure that any non-illuminated parts reset to being hidden.
You should see something that looks like this:
Next Steps
You now have the tools to create a flashlight or spotlight effect that can be used to reveal things shrouded in darkness!
What else you can do with this technique is up to your imagination!
A pitch-black Scene can be explored by flashlight if you don't use a cover image at all. Maybe there's a horror game here somewhere? 🤔
Let us know in the comments below if anything doesn’t work or is unclear. We’ll be happy to fix or clarify!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
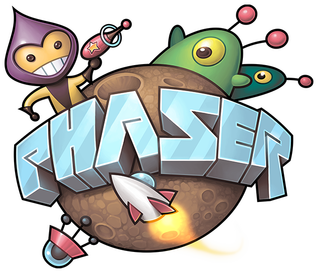