JavaScript is great for creating small games but have you ever gone back to an old JavaScript project and had no idea what properties exist on an object or what should be passed to a function?
The only way to figure out what's going on is to console.dir
objects or use the debugger. And even then you won't easily figure out what properties are optional or required at a later point.
The odds of introducing a null reference exception or other bug is very high in this state.
There are a few ways to solve this problem:
- use JSDoc to annotate your objects and functions
- use a type checker like Flow
- use TypeScript: a typed superset of JavaScript
All three options are good and in this article, we are going to look at using TypeScript to make your game code more scalable and maintainable.
Note: you can uses tests to mitigate some of the potential issues as well but it won't catch them while you are writing the code.
Simplicity with Parcel
There are many ways to set up a Phaser 3 project with TypeScript depending on what bundler you use or if you decide to hand-roll your setup.
We recommend using Parcel and the phaser3-parcel-template.
Check out our guide to using the template here if you need detailed instructions. Otherwise clone the template to follow along:
git clone https://github.com/ourcade/phaser3-parcel-template.git my-folder-name
cd my-folder-name
npm install
Add a tsconfig
This step is not strictly necessary but it is recommended to support absolute paths, change TypeScript compiler settings, or configure your code editor. Here's a recommended tsconfig.json
file. Create it in the project root.
|
|
Learn more about <code>tsconfig</code> options here.
Using TypeScript
There is no other set up steps thanks to Parcel! 🎉
The only thing you need to do is change the .js
files to .ts
and start writing TypeScript.
Here's what we did to main.ts
as an example:
|
|
Notice that we are giving config
a type of Phaser.Types.Core.GameConfig
on line 5. Now you'll get property suggestions with a code editor like VS Code.
Bouncing Phaser Logo
We will move the basic bouncing Phaser logo logic into a class to demonstrate some more TypeScript features!
|
|
Here we have a wrapper class that creates the physics image and sets the physics properties in initialize()
. We are using the private
modifier on a class property and a class method.
We are also specifying type in the constructor
arguments. Now you'll always know that texture
is a string
and not something else like a Texture
instance.
Putting it Together
To put it all together we will convert the HelloWorldScene
to use TypeScript features and the BouncingLogo
we created.
|
|
TypeScript supports enums and we create one on line 4 in place of constant variables for unique image names. In modern JavaScript you would use something like: const ImageNameSky = 'sky'
.
Then we create our BouncingLogo
on line 32 using the ImageNames.Logo
enum.
Last thing of note is the private createEmitter(textureName: string)
method that has the private
access modifier and a typed textureName
parameter.
You can test all this out if you haven't already by going into your project folder and using:
npm run start
Open http://localhost:8000 in your browser and the familiar Phaser logo will be bouncing around. 🎉
Next Steps
Using TypeScript with Phaser 3 is a piece of cake when you use Parcel and the phaser3-parcel-template.
You can use all TypeScript features from decorators to generics. Learn more about TypeScript here.
Let us know in the comments below if anything doesn’t work or is unclear. We’ll be happy to fix or clarify!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
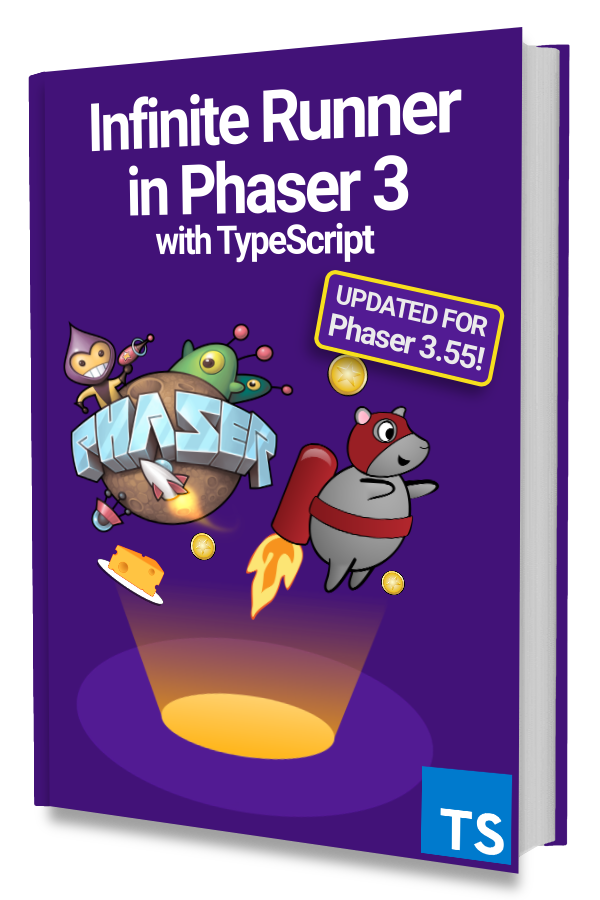