Are using modern JavaScript and splitting your Phaser 3 game code into multiple files? 👏
One common best practice is to put the UI code in a separate Scene.
But then how are you supposed to give the UI Scene information like player health or score?
How would a pause button in the UI Scene tell the Game Scene to pause?
One solution is to pass data using events.
The easiest way to implement this is to use the EventEmitter
instance from this.game.events
but…
You should generally avoid accessing any of the systems created by Game, and instead, use those made available to you via the Phaser.Scene Systems class instead.
🤔
No problem! We can make our own EventEmitter
instance to use!
It is super easy and we'll show you how in this article.
Creating an EventEmitter
Every Scene has an events
property that is an EventEmitter
instance local to each Scene.
It is generally used to listen for Scene events like SHUTDOWN
to perform any cleanup.
Since each Scene has a local instance, it can't be used to pass data between two different Scenes.
There is an EventEmitter
instance available in the Game
instance but accessing this.game
is generally not recommended.
You also risk emitting event names used by Phaser when you use this.game.events
. This can result in undefined behavior.
Your safest bet is to make an EventEmitter
instance that no one else is using.
We can do that by creating a new file named EventsCenter.js
like this:
|
|
Just 3 lines of code! It can even be turned into 2.
Now let's see how we can use it in two Scenes.
Communicating Between Two Scenes
Our example will have a simple UIScene
class that can update a Text
object to show a counter.
|
|
Then in our Game
Scene, we update a count
property each time the space
key is pressed.
This is what create()
looks like:
|
|
First, we import the eventsCenter
instance on line 2.
Then we run the UIScene
in parallel with the Game
Scene on line 9.
The important part is line 14 where we emit an event named 'update-count'
with the current value of this.count
.
At this point, UIScene
is not listening to any events from eventsCenter
.
Let's change that by updating create()
in UIScene
:
|
|
Pressing the space
key will trigger the count
property in the Game
Scene to increment and emit an event called 'update-count'
.
Then the UIScene
will update the Text
object to show the new value of count
.
It will look something like this:
Next Steps
Now you can easily create multiple Scenes that run in parallel and have them pass data to each other.
The example above just shows communication from Game
to UIScene
but you can just as easily pass information in the other direction for a pause button.
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
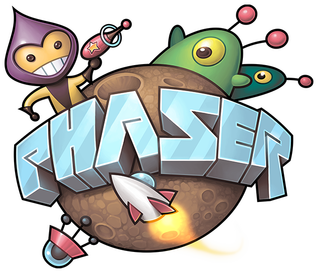