Does your game feel too static or not reactive enough?
Do coins just disappear when the player collects them?
There's just something unsatisfying about that interaction, right?
A greater feeling of liveliness would be beneficial and particles are a great way to add it!
In this article, we will look at using a particle trail from a collected coin to the score counter in the UI.
We'll be using the Rocket Mouse game from our Infinite Runner in Phaser 3 with TypeScript book.
You can get it for free by dropping your email into the box below. It is a great resource to have if you are looking to use TypeScript with Phaser 3!
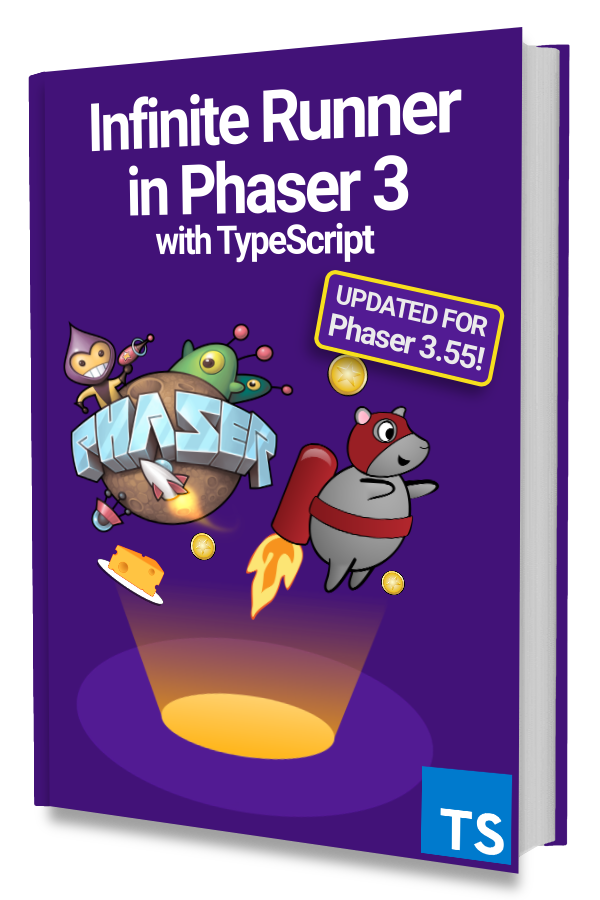
Learn to make an Infinite Runner in Phaser 3 with TypeScript!
Drop your email into the box below to get this free 90+ page book and join our newsletter.
Create a ParticleEffects Scene
The Rocket Mouse infinite runner game is always scrolling but the particle trail effect that we will be making plays in the UI space that should not scroll with the game environment.
We can try to calculate offsets based on the camera's scrollX
property but it would be much simpler to create a new Scene for the particle trails.
So let's create a ParticleEffects.ts
file in the scenes
folder like this:
|
|
Notice that we also added a new enum
member to SceneKeys
that should look like this:
|
|
Next, let's add the ParticleEffects
Scene to the scene
list in our GameConfig
:
|
|
Now, we can run this Scene as soon as the Game
Scene starts in the init()
method like this:
|
|
Add a create()
method to the ParticleEffects
Scene with a console.log('hello!')
to make sure it is being run.
Send a Message on Coin Collect
The goal is to create a particle trail that goes to the score counter each time a coin is collected and keep all that particle logic contained.
To achieve this goal we will pass a message to the ParticleEffects
Scene in the handleCollectCoin()
method.
Let's do that by getting a reference to the ParticleEffects
Scene and then emitting an event.
|
|
A reference to the ParticleEffects
Scene is retrieved with this.scene.get(SceneKeys.ParticleEffects)
and stored in particleEffects
.
Then we emit a 'trail-to'
event using the Scene's EventEmitter
with data about where the trail should start from and go to.
The main camera's scrollX
is used to offset the fromX
value and then the toX
and toY
values are calculated based on where this.scoreLabel
is.
Next, we have to listen for the 'trail-to'
event in the ParticleEffects
Scene and create the particle trail effect.
Creating a Particle Trail
We've added a star.png
file to use as particles. Pick an image of your own and preload it like this:
|
|
We are using the enum
value ParticleStar
. Remember to add that to TextureKeys
like this:
|
|
Next, we need to create a ParticleEmitterManager
. Let's do that in the create()
method like this:
|
|
Then, listen for the 'trail-to'
event and create a ParticleEmitter
:
|
|
The important new code is on lines 15 - 27 where we listen for the event and create a ParticleEmitter
. The fromX
and fromY
values are used as the starting point and then the rest of the properties can be adjusted for a different look and feel.
We also added an interface
called TrailToData
to help us with typing information in the event callback.
Try the game now and you'll see particles created in place of a collected coin.
To animation the particle trail we will create a counter tween that generates values to move along a Catmull-Rom spline.
|
|
First, we create 2 arrays to hold the positions for the Catmull-Rom spline to use called xVals
and yVals
.
Then we create a counter tween with this.tweens.addCounter()
that goes from 0
to 1
with a duration of 1
second using a Sine.InOut
ease.
For each onUpdate()
we calculate the Catmull-Rom position at the given value between 0
and 1
. The calculated position is then used to move the emitter
to the score counter UI.
When the counter tween is finished, we perform a particle explosion and remove the emitter after 1
second. This cleanup step is important since we are creating a new ParticleEmitter
each time the 'trail-to'
event is fired.
The effect should look something like this:
Final Cleanup
The last thing we need is to stop listening for the 'trail-to'
event when the ParticleEffects
Scene is shutdown.
|
|
Next Steps
Particle trails are an effective way to help players read a user interface by pointing out meters that relate to a collectible.
It also adds a feeling of liveliness and some extra eye candy. ✨
Try adjusting the values in the ParticleEmitter
config and the ones given to the Catmull-Rom spline to achieve a different look & feel!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
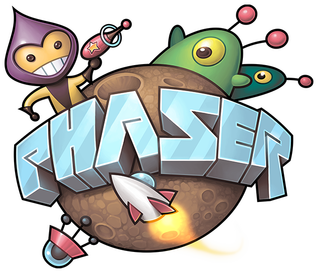