Games can be some of the most complicated types of software you can create. And the most fun.
It helps to use modern best practices to avoid messy bugs or more easily fix ones that come up.
Doing so saves you time and headaches. Most importantly it will help you make a better game.
In this article, we will show you how to create games in Phaser 3 using modern JavaScript and best practices.
We'll be creating the same game from the official Making your first Phaser 3 game guide. This way you can compare and contrast the differences.
In Part 1 we created a new project and added a GameScene. Go check it out if you haven't already.
Creating Platforms
To add platforms and ground to our game we will use the ‘ground’ asset we preloaded in Part 1.
The code will be placed in a method instead of being added to the create()
method. Organizing code into reusable functions and methods helps with writing better code.
|
|
The line to add the star has been commented out (line 10 above) to show that we no longer need it. You can delete it.
The platform creation code is the same as the official Phaser guide except we've organized it to be more readable. Check part 4 of the official guide if you want to understand how the code works.
You should see green platforms added to your game at http://localhost:8000. It should refresh automatically if you left the browser open from Part 1.
Don't Repeat Your Strings
You may have heard of the DRY Principle of software engineering. It is a good one to follow.
We are going to apply it to our string literals.
You can see that we used the string 'ground'
4 times in createPlatforms()
and 1 time in preload()
.
As humans, we tend to make typos. The best way to avoid typos is to avoid typing. 😂
We can avoid typing by using a constant variable.
|
|
The benefits you get using a constant variable is autocompletion by your code editor–no typing need!–and clear errors if you misspell the variable name. 👍
Create a Player
The player will be created using the dude.png
sprite sheet that was loaded in preload()
. The player creation code is placed in a createPlayer()
method like we did for platforms.
|
|
Notice that we applied DRY to the 'dude'
string literal with the DUDE_KEY
constant.
We added a player
instance property and set it to undefined
in the constructor. This is not strictly necessary. Declaring all instance properties before using them makes it easier to see all the properties are at a glance.
The player instance is later created in the createPlayer()
method which is called from create()
.
The page at http://localhost:8000 should automatically refresh and show your player falling to the bottom of the screen.
Coming Up
We've now transformed parts 3 - 5 from the official Making your first Phaser 3 game guide into modern JavaScript.
Let us know in the comments below if anything doesn’t work or is unclear. We’ll be happy to fix or clarify!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
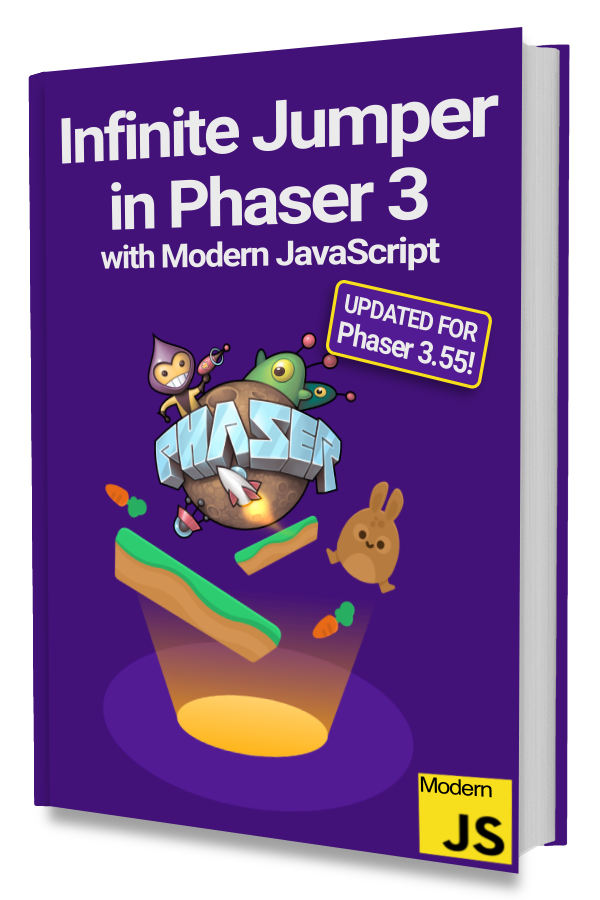