Games can be one of the most complicated pieces of software you create. And the most fun.
It helps to use modern best practices to avoid messy bugs or more easily fix ones that come up.
Doing so saves you time and headaches. Most importantly it will help you make a better game.
In this article, we will show you how to create games in Phaser 3 using modern JavaScript and best practices.
We'll be creating the same game from the official Making your first Phaser 3 game guide. This way you can compare and contrast the differences.
In Part 2 we added platforms and created a player character. Go check it out if you haven't already.
Colliders
We have platforms and a player but they are not colliding with each other. Let's fix that by adding a collider for the player physics body and the platform static group.
|
|
The collider is added on line 17 but we made more changes.
The createPlatforms()
and createPlayer()
methods now return the instance they are creating.
It is a best practice to return values for functions with names like create...
, make...
, construct...
, etc. This makes for clearer and safer code as opposed to the function changing variables outside its scope.
Our createPlayer()
method was previously changing this.player
in the class scope. Now it creates a local variable and returns it without making unseen changes or side effects.
You generally want your functions to be as pure as possible. Some of the worst bugs are due to unintended mutations or side effects.
Keyboard Controls
Now that we've added a collider our player is interacting with the platforms as we expect.
Next, we will add some keyboard controls.
|
|
We added a cursors
property on line 12 and then set it to Phaser's default cursor keys in the create()
method on line 26.
The new update()
method is where the input logic takes place. The code is the same as the official Phaser guide except we are using references to class properties (this.cursors
and this.player
) instead of globals.
Your browser window at http://localhost:8000 should automatically refresh. Then you can move your character with the left/right arrow keys and jump with the up key! 🎉
Coming Up
We've now transformed parts 6 and 7 from the official Making your first Phaser 3 game guide into modern JavaScript.
In the next part, we will add stars to collect and handle showing a score.
Let us know in the comments below if anything doesn’t work or is unclear. We’ll be happy to fix or clarify!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
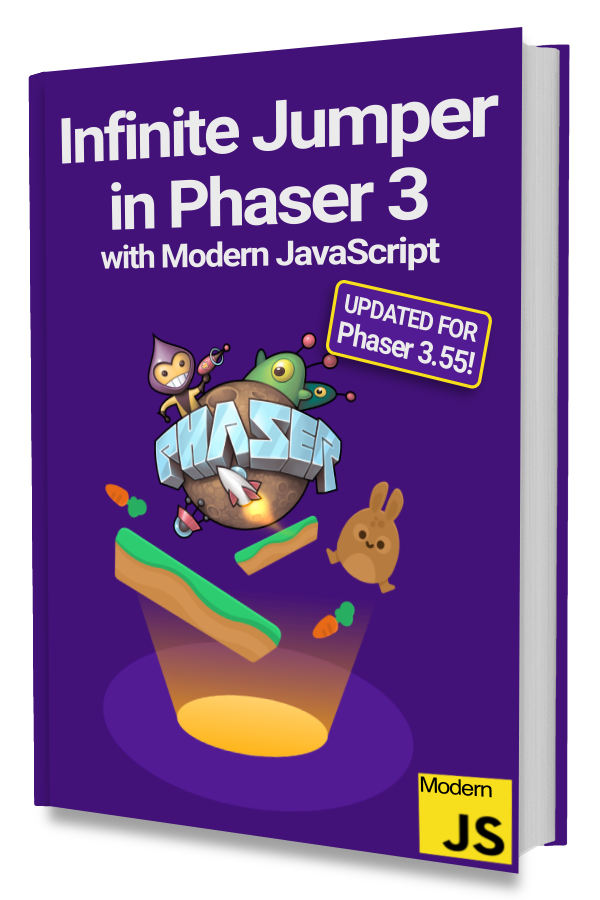