Are you working on a side-scrolling platformer in Phaser 3 using Matter Physics?
And are you having trouble implementing moving platforms? Ones that can be used as elevators or add some extra challenge to horizontal jumps?
Matter has some quirks that can make creating moving platforms a frustrating experience.
That's where this article comes in! We'll show you how to create moving platforms that can carry the player up and down or across pits.
Example Set-Up
We'll be using modern JavaScript in this example as well as platformer assets from Kenney.
If you are not familiar with using modern JavaScript with Phaser 3 then we have a free book to help you get started!
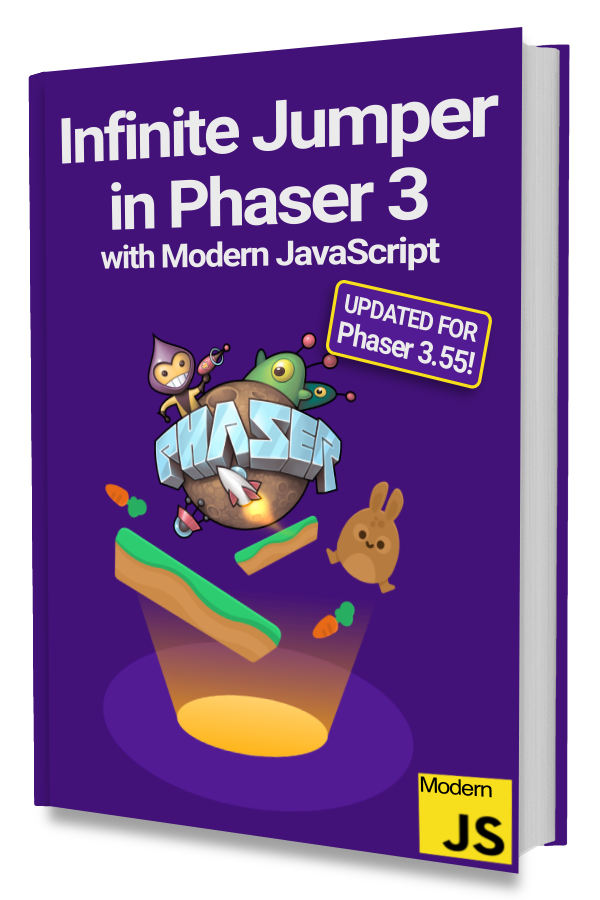
Learn to make an Infinite Jumper in Phaser 3 with modern JavaScript!
Drop your email into the box below to get this free 60+ page book and join our newsletter.
Our focus will be on implementing a MovingPlatform
class that can move vertically or horizontally. We assume that you already have a working player character that can run and jump.
The MovingPlatform Class
First, let's create a barebones MovingPlatform
class that extends from Phaser.Physics.Matter.Image
:
|
|
Next, let's create an instance of this class in our Scene.
|
|
The key here is that we need to make the platform static by passing in isStatic: true
as the MatterBodyConfig
.
A static
body will not move or be affected by gravity but it will collide with other non-static bodies in the world. This will allow us to manually move the platform up and down or left and right.
In most platformers, a moving platform travels a predefined distance and then returns. It is much simpler to control the platform's movement manually than to adjust the velocity depending on where the platform should travel to.
Moving the Platform Vertically
Phaser comes with a tween system that can be used to move our platform up and down over time with easing.
Let's add the logic by creating this moveVertically()
method to the MovingPlatform
class:
|
|
Notice that we are using addCounter()
. This will tween a number from 0
to -300
as specified on lines 4 and 5. We then use the tweened value in the onUpdate
callback.
Setting this.y
and then setting the y
velocity is important. Other bodies on the platform will not move with it unless the velocity is set.
We also set yoyo
to true
and repeat
to -1
so that it will move up then back down and loop forever.
An additional class property named startY
was created and it should be set to the initial y
value in the constructor:
|
|
Try out the vertically moving platform by calling moveVertically()
after creating the platform.
|
|
You should get something that works like this:
Moving the Platform Horizontally
Now that we have vertical movement, let's implement horizontal movement.
The basic concepts will be the same except we have to adjust the platform's friction or bodies on the platform may not stay there as it moves.
Adjust the platform's friction in the constructor:
|
|
Notice the call to setFriction()
where we pass in Infinity
for the staticFriction
argument.
We also created a class property named startX
to store the initial x
value on line 9.
Next, let's add a moveHorizontally()
method:
|
|
This method is almost identical to moveVertically()
except we adjust this.x
and the x
velocity for horizontal movement.
Try it out by calling moveHorizontally()
after creating the platform.
Next Steps
You can add duration or distance parameters to the moveVertically()
and moveHorizontally()
methods to allow different platforms to move at different speeds and distances.
Feel free to play with the ease
property to get a different type of movement.
With the techniques from this article, you should be able to make diagonally moving platforms as well!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
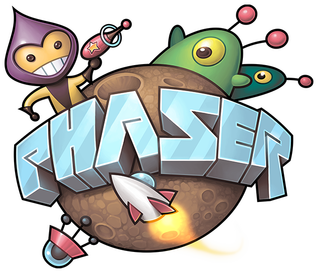