If you've gone through the basic first game in modern JavaScript or the infinite jumper book then you've got the basics down so let's try making something a little bit more complicated!
We suggest Memory Match. A Mario Party-inspired memory game where you control a character to pick boxes until all matches are found within a limited amount of time.
For this part 5, we'll add logic that stuns the player when the bear is revealed and then check for level complete when all matches have been made.
We also have a video version on YouTube if you prefer to watch or want to see how it is coded in real-time.
Stay Away From Bears
In Mario Party's memory match, Mario becomes stunned and unable to move after selecting a tile with Bowser behind it.
We'll do something similar by disabling the player from moving when the box with a bear in it is opened.
Let's start by adding a check after the tween that reveals an item is finished. Add this to the onComplete
callback in the openBox()
method:
|
|
We check to see if itemType
is 0
which represents the bear. If true, we call handleBearSelected()
and early exit.
Next, let's implement handleBearSelected()
:
|
|
First, we call pop()
as we did in the previous part to get the last selected box and item. We are using modern JavaScript's Destructuring Syntax on line 4.
It is simply a shorthand for this:
|
|
Then we add a red tint to the bear and change the box to use frame index 7
which is a red box.
Next, we set the player to inactive and stop all movement on lines 13 and 14.
After that, we wait 1 second before restoring everything to normal and tweening the bear out like other items.
We have to add one more thing to stop the player from moving when active
is set to false
. Add this to updatePlayer()
:
|
|
Now when you open a box with the bear, it will turn red and the player will be unable to move for 1 second. 😎
Checking for Level Completed
You can basically play the entire game now except nothing happens after you find all the matches.
We can do this by keeping a count of how many matches we've found and then compare it against the total matches that can be found in this level.
There are 9 boxes and 1 is the bear which is just there to slow the player down. The remaining 8 make up 4 matching pairs. This means the level is completed when 4 matches are found.
Let's turn that logic into code by first, adding a matchesCount
property to the Game
Scene:
|
|
Notice that we don't need to specify a type using JSDoc because we are setting it to a number.
Next, we want to increment matchesCount
each time a match is found. We can do that in checkForMatch()
like this:
|
|
Now we need to check when it is equal to 4 and show a “You Win” message. The player should be disabled as well.
We can do that right after the boxes are changed to use frame index 8
which is a blue box showing that a match has occurred.
|
|
First, we add a check to see when this.matchesCount
is at least 4 on line 12.
When that is true, we disable the player as we did for revealing the bear and then add a “You Win!” text on line 22.
If you would like to make nicer looking text then we recommend taking a look at our Text Styler for Phaser 3 tool. You'll also want to check out this tutorial on loading fonts from Google Fonts here.
Find all the matches and you should see something like this 👇
Next Steps
Our mini Memory Match game is almost done! We can walk around, get stunned, make matches, and be notified when we've completed the level.
The last thing we have left is to add a countdown timer so that there is some challenge. That's what we'll do in part 6!
Be sure to sign up for our newsletter so you don't miss any future Phaser 3 game development tips and techniques!
Drop your email into the box below.
Don't miss any Phaser 3 game development content
Subscribers get exclusive tips & techniques not found on the blog!
Join our newsletter. It's free. We don't spam. Spamming is for jerks.
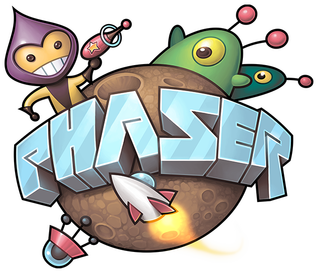